Programming basics
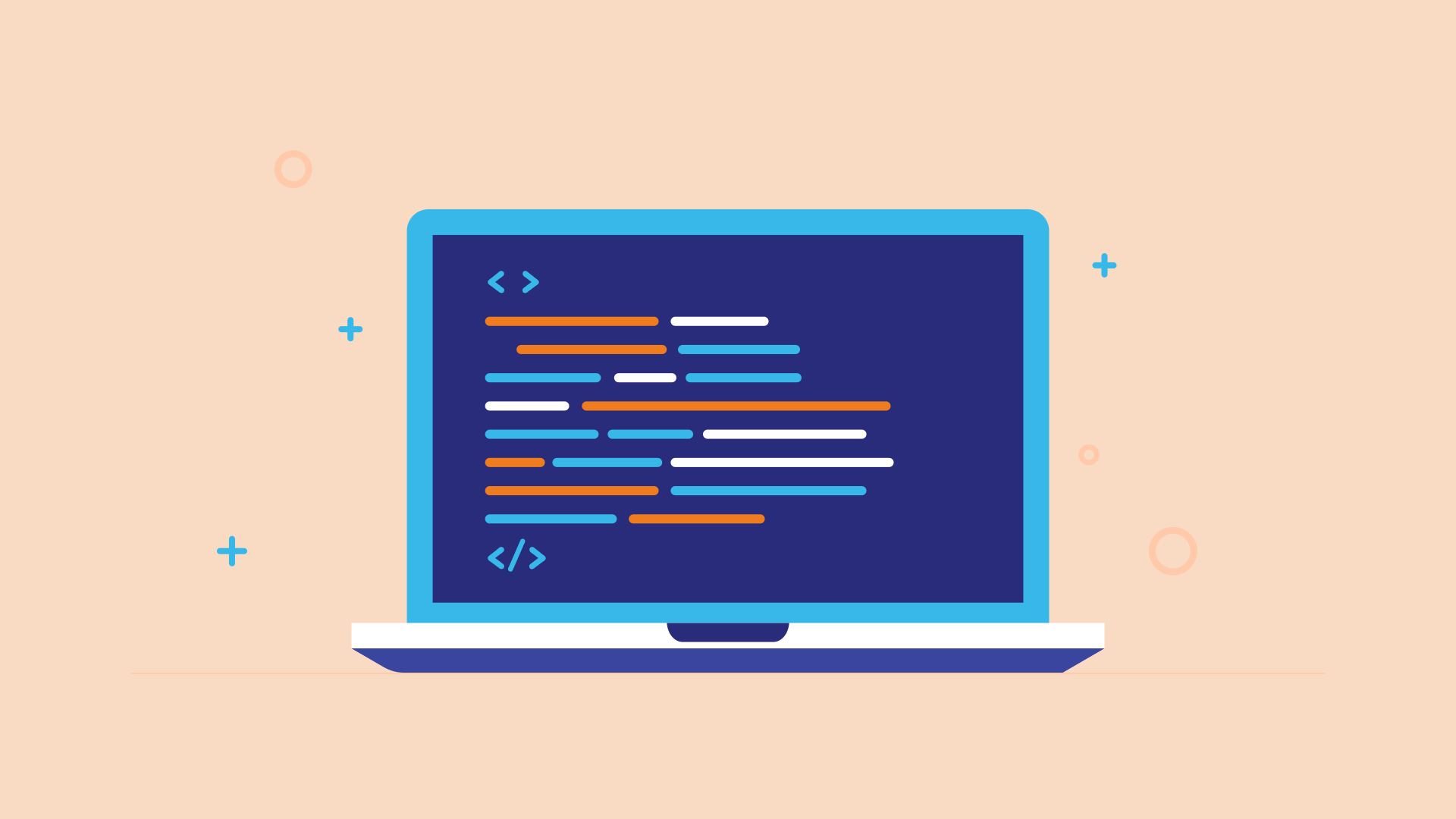
Computer programs are used by people every day. Everytime you use your smartphone, tablets, and laptops, all the apps are computer programs. When you watch a movie on Netflix or Amazon, or even driving a Tesla, you are also using a program.
A computer program consists of basic instructions
- Input: A program gets data – typically from a file, keyboard, network, or touchscreen
- Process: A program performs computations on the data, such as multiplying two values like a * b.
- Output: A program outputs the data – typically on the screen, to a file, database, or network
Programs use variables to refer to data, such as name to hold a person’s name, or age for person’s age.
An algorithm is sequence of instructions for, solving a problem. For example, an algorithm can be the steps for making a sandwich to eat, or the steps for calculating the distance between two GPS points.
Programs are written using a high-level programming language such as Python, Java or C++.
Input and output
To print output to the screen, use the print() function. Ex: print('hello world’).
The text ‘hello world’ is called a string literal. A string literal may contain any characters such as letters, numbers or symbols such as $ or #. In python, you can use either single or double quotes to surround a string literal. print(‘hello world’) and print(“hello world”) both print the text hello world to the screen. If text represents numbers such as 187, or 11.99, then there are no quotes around the numbers. A string ‘187’ is different than a number 187 in Python. 187 represents the number 187, while string ‘187’ is a character sequence ‘1’, ‘8’ and ‘7’.
If you need to print out a combination of string literals and variables, separate each item with a comma. Ex:
age = 20
print('Your are', age, 'years old.') # Comma separates multiple items with a space
print('Have a nice day!')
Outputs:
You are 20 years old.
Have a nice day!
Calling the print() function outputs on a new line. If you want to keep output on the same line., then add end=' ' inside of print() to separate the next line by a single space. Ex: print('Hello', end=' ‘).
You can print out the newline character ‘\n’ whenever you need to output a new line. Ex: print(‘A\nB\nC’) outputs:
A
B
C
A whitespace refers to any space, tab, or newline in your program. Whitespace matters in Python, as you will see soon as you learn more python.
Programs may allow a user to enter input values, such as a number or string. In python, you can read input using the input() function. Ex: name = input() will set the variable name to the text entered by the user.
Any data read by the input() function is read in as a string, so if you wanted to convert string ‘187’ to the integer 187, then use the int() function. Ex:
some_int = int(‘187’)
Types of errors
Syntax errors
Everyone makes mistakes, and one of the most common Python mistakes is a syntax error, which is invalid code that the python interpreter doesn’t understand. The interpreter finds syntax errors before you are able to run the program, and gives you some hints on how to fix them. It takes some practice dealing with syntax errors, but eventually you should get the hang of it and fix them quickly. Ex:
print(name); # should not have a semicolon at the end
print("hello world') # wrong closing quote
It’s good practice to just write a few lines of code, run it and verify that it works, then write a few more lines of code.
Runtime errors
A runtime error is an error that crashes your program while it is running. The Python interpreter does not detect a runtime error because the program's syntax is correct but the program attempts an illegal operation, such as dividing by zero or multiplying strings literals. When a runtime error happens, your program crashes and halts execution. Ex:
print('hello' * world)
print('the end')
Logic errors
Making a logical mistake in your code, such as multiplying when you should have added is a logic error. The interpreter doesn’t detect logic errors, and the program also doesn’t produce a runtime error and crash. Your program simply just produces the wrong output. Ex:
sum = 3 * 4
print('the sum of 3 and 4 is', sum)
Other common types of errors
Indentation error is when you didn’t properly indent your code. Ex:
print('why did I indent this?')
Value error is when you pass the wrong value to a function such as int(). Ex:
int('five') # cannot pass letters to int(), only number string literals
Name error is when the program tries to use a variable that does not exist. Ex:
first_name = 'Bob'
print(last_name)
Type error is when an incorrect type is used, such as adding a string to an integer. Ex:
age = 20 + ' years old'
- Technology
- Ausbildung
- Business
- Music
- Got talent
- Film
- Politics
- Food
- Spiele
- Gardening
- Health
- Home
- Literature
- Networking
- Other
- Party
- Religion
- Shopping
- Sports
- Theater
- Wellness