Variables, Assignments, and Identifiers
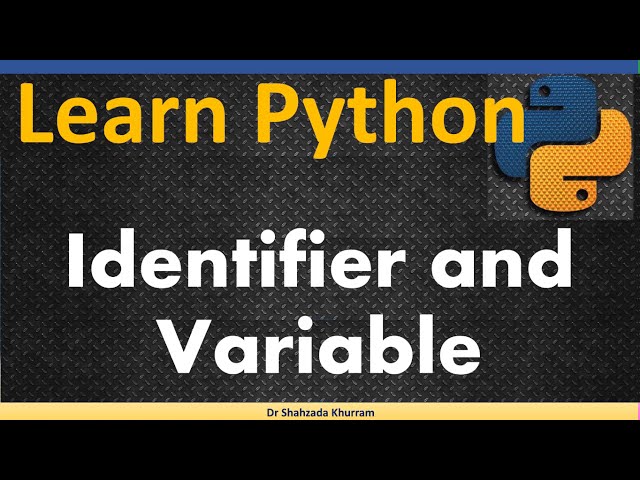
Variables
Variables are used to store data that can be used and manipulated throughout your program. In Python, you don't need to declare the type of a variable; it is dynamically typed.
Assignments
Assignment is the process of storing a value in a variable. The assignment operator in Python is the equals sign (=
).
Identifiers
Identifiers are names given to variables, functions, classes, etc. They must follow certain rules:
- Must begin with a letter (a-z, A-Z) or an underscore (
_
) - Can contain letters, digits (0-9), and underscores
- Case-sensitive (e.g.,
myVar
andmyvar
are different) - Cannot be a reserved keyword in Python (e.g.,
if
,else
,for
, etc.)
Examples
Variable Assignment
# Assigning values to variables
x = 10
name = "Alice"
pi = 3.14
Using Identifiers
# Valid identifiers
age = 25
first_name = "John"
_number = 42
# Invalid identifiers (uncommenting these lines will cause an error)
# 1st_variable = 10 # Cannot start with a digit
# my-variable = 20 # Hyphens are not allowed
# class = "Math" # 'class' is a reserved keyword
Reassigning Variables
You can reassign variables to new values, and even change their types:
x = 10 # x is an integer
x = "Hello" # x is now a string
Best Practices
- Use meaningful variable names to make your code readable and maintainable.
- Follow the PEP 8 style guide for Python code, which recommends using lowercase letters and underscores for variable names (
snake_case
).
By understanding and using variables, assignments, and identifiers correctly, you can write clear and efficient Python code.
- Technology
- Educação
- Business
- Music
- Got talent
- Film
- Politics
- Food
- Jogos
- Gardening
- Health
- Início
- Literature
- Networking
- Outro
- Party
- Religion
- Shopping
- Sports
- Theater
- Wellness