Tuple, Set, and Dictionary
Сообщение 2024-07-16 21:35:59
0
5Кб
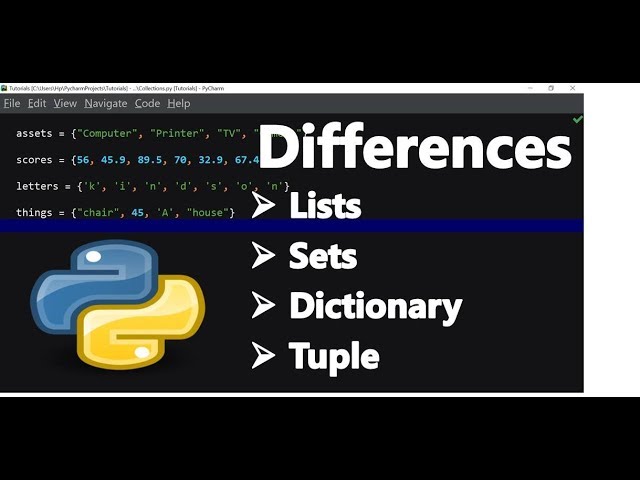
These are all fundamental data structures used to organize information in programming. Here's a breakdown of each:
Tuple:
- Ordered collection of elements, similar to a list.
- Elements can be of different data types (strings, numbers, etc.).
- Immutable: Once created, you cannot change the elements within the tuple.
- Used for representing fixed data like coordinates (x, y) or product details (name, price, stock).
Set:
- Unordered collection of unique elements.
- Elements can be various data types.
- Useful for storing unique items and performing set operations like checking membership or finding differences between sets.
Dictionary:
- Unordered collection of key-value pairs.
- Keys must be unique and immutable (often strings or numbers).
- Values can be any data type.
- Used for storing data where you need to access it by a specific key, like phone numbers in a phonebook (key: name, value: phone number).
Here's a table summarizing the key differences:
Feature | Tuple | Set | Dictionary |
---|---|---|---|
Order | Ordered | Unordered | Unordered |
Mutability | Immutable | Mutable | Mutable |
Duplicate Data | Allowed | Not Allowed | Not Allowed (for Keys) |
Access Method | By index | By membership | By key |
Поиск
Категории
- Technology
- Образование
- Business
- Music
- Got talent
- Film
- Politics
- Food
- Игры
- Gardening
- Health
- Главная
- Literature
- Networking
- Другое
- Party
- Religion
- Shopping
- Sports
- Theater
- Wellness
Больше
NEW CURRICULUM CHEMISTRY PRACTICAL SAMPLES
https://acrobat.adobe.com/id/urn:aaid:sc:EU:4838a935-5393-417a-a9b9-a4c21d6109cb
Turkish Airlines Flight Makes Emergency Landing at JFK After Pilot Dies
New York, October 9, 2024 – A Turkish Airlines flight from Seattle to Istanbul made an...
MODERN AFRICAN NATIONAL HISTORY MADE EASY
https://acrobat.adobe.com/id/urn:aaid:sc:EU:4838a935-5393-417a-a9b9-a4c21d6109cb
Intro to Classes and Objects python Show drafts
Classes: The Blueprints
Imagine you're building a house. You wouldn't just start hammering and...
The Ten Commandments of Computer Ethics
The Ten Commandments of Computer Ethics were created by the Computer Ethics Institute to guide...