Operators and Precedence Rules
In Python, operators are special symbols that perform operations on operands (values or variables). Operator precedence determines the order in which these operations are evaluated within an expression. Understanding precedence is essential for writing correct and predictable code.
Precedence Levels:
Python follows the PEMDAS (or BODMAS) order of operations, just like basic math. Here's a breakdown from highest to lowest precedence:
- Parentheses
()
: Expressions within parentheses are evaluated first. You can use parentheses to override the default order or to group subexpressions. - Exponentiation
**
: This operator performs powers or exponentiation. For example,2 ** 3
equals 8. - Multiplication and Division (
*
,/
,%
): These operators have equal precedence. Multiplication and division are evaluated from left to right. The modulo operator (%
) calculates the remainder after a division. - Addition and Subtraction (
+
,-
): Similar to multiplication and division, these operators have equal precedence and are evaluated left to right.
Example:
expression = 2 + 3 * 4 - (10 / 2) ** 2
Following PEMDAS:
- Evaluate the expression within parentheses first:
(10 / 2) ** 2 = 5 ** 2 = 25
- Perform exponentiation:
2 + 3 * 4 - 25
- Multiplication:
2 + 12 - 25
- Left-to-right addition and subtraction:
-11
Associativity:
When multiple operators have the same precedence level, associativity determines the direction of evaluation (left to right or right to left). Almost all operators in Python are left-associative, meaning they are evaluated from left to right. However, the exponentiation operator (**
) is an exception - it's right-associative.
Example:
expression = 2 ** 3 ** 2 # Right associativity for **
This is evaluated as 2 ** (3 ** 2)
, which equals 2 ** 9
or 512.
Tips for Using Operators:
- Use parentheses liberally to improve code readability and enforce the desired evaluation order, especially in complex expressions.
- Be mindful of the precedence and associativity rules to avoid unexpected results.
- If the order of evaluation is unclear, break down the expression into smaller steps or use parentheses for clarity.
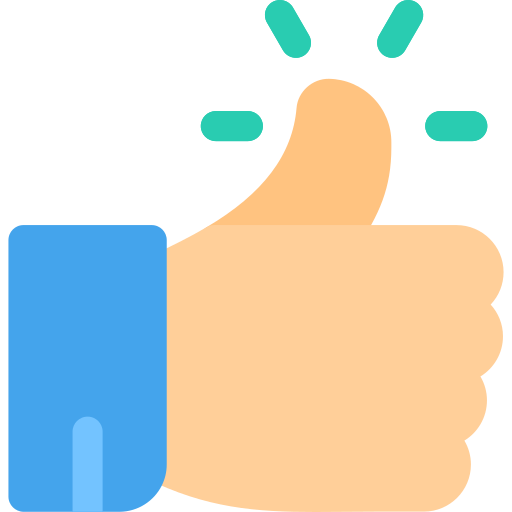
- Technology
- Formazione
- Business
- Music
- Got talent
- Film
- Politics
- Food
- Giochi
- Gardening
- Health
- Home
- Literature
- Networking
- Altre informazioni
- Party
- Religion
- Shopping
- Sports
- Theater
- Wellness