Functions, Parameters, and Return Values in python
Posted 2024-07-17 14:52:00
0
5K
Python uses functions extensively! Here's how functions, parameters, and return values work in Python:
Defining Functions:
In Python, you define functions using the def
keyword followed by the function name and parentheses:
Python
def function_name(parameter1, parameter2, ...):
# Function body (code to be executed)
return value # Optional return statement
function_name
: This is the name you choose for your function.parameter1, parameter2, ...
: These are comma-separated variables that act as placeholders for the data you'll provide when you call the function.
Calling Functions:
You call a function by using its name followed by parentheses:
Python
result = function_name(argument1, argument2, ...)
argument1, argument2, ...
: These are the actual values you provide to the function, corresponding to the defined parameters.- The result of the function (if it returns a value) is assigned to a variable (here,
result
).
Return Values:
- Functions can optionally return a value using the
return
statement. This value is sent back to the code that called the function. - The
return
statement can return any valid Python object (numbers, strings, lists, etc.).
Example:
Here's a Python function that calculates the area of a rectangle and returns the value:
Python
def calculate_area(length, width):
"""Calculates the area of a rectangle."""
area = length * width
return area
# Calling the function and using the return value
rectangle_area = calculate_area(5, 3)
print(f"The area of the rectangle is: {rectangle_area}")
This example demonstrates:
- Defining a function with comments (using triple quotes).
- Using parameters (
length
andwidth
). - Calculating the area within the function body.
- Returning the calculated area using
return
. - Calling the function and storing the returned value (
rectangle_area
).
Key Points:
- Parameters allow you to provide custom input to your functions.
- Return values give functions a way to send data back to the calling code.
- Functions promote code reusability, modularity, and maintainability.
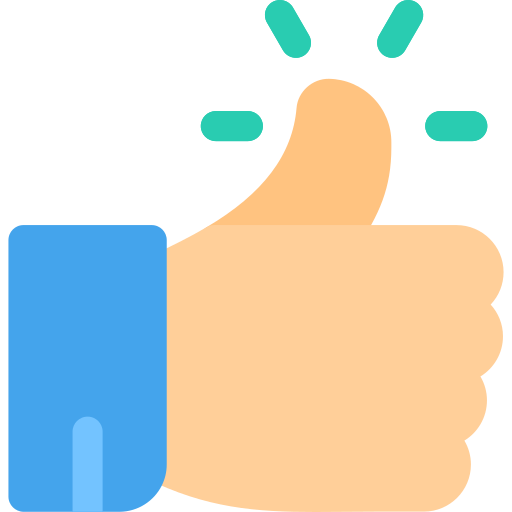
Cerca
Categorie
- Technology
- Formazione
- Business
- Music
- Got talent
- Film
- Politics
- Food
- Giochi
- Gardening
- Health
- Home
- Literature
- Networking
- Altre informazioni
- Party
- Religion
- Shopping
- Sports
- Theater
- Wellness
Leggi tutto
Line breaks (<br>)
The <br> tag is used to insert a single line break in your HTML content. It's an empty...
Scenarios of Computer Misuse and Their Effects on Society
Computer misuse can have widespread and serious impacts on society. It encompasses a wide range...
Basic structure of an HTML document
An HTML document is composed of two main sections: the head and the body.
The <head>...
CBTI UCE BIOLOGY MOCK EXAM PAPER 1
CBTI UCE BIOLOGY MOCK EXAM PAPER 1
Understanding the SUMPRODUCT Function
The SUMPRODUCT function in Excel is a powerful tool used to perform array-based calculations...